
Main reference:
Getting started:
Build modules on the browser including non-js file
$ webpack <entry.js>
$ webpack-dev-server –port=9000
Entry point
a module relys on other module, dependncies
the first js to load to ‘kick-off’ your app, a starting point for webpack to build the dependency graph
what to load for the browser; compliments the output property.
Output
where and how to distributes the bubdles (compilations)
default: bundle.js
Loaders
js functions that takes the source fiel, and return a modifed state
tell webpack how to load non-js file(transfer & interpretation)
return compliations
test
RegEx instructs the complier which files to run the loader against
use
An array/string/function which returns loader objects
enforce
Pre/post
run this rules before/after all other rules
include&exclude
Filter through
eg.node-modules
chaining loaders
order sensitive
like a streamline
Plugin
a class/instance which has an ‘apply’ method which can be used by complier to emit event
1 | examplePlugin.protyotype.apply= funciton(complier){ |
To use:
require() plugin from node_modules into config
add new instance of plugin to plugin keys in config object
provide additonal info for arguments
Tapable
backbone of webpack plugin
mixin
Apply plug asyn / parallel
Event emitter/hook
1 | class basicPlugin { |
1 | class somePlugin{ |
the plugin is registerd via compliers inherited apply function
1 | class Compiler extends Tapable{ |
Tapable instances call apply.plugins() and pass the event and state through to plugin
.run() -> trigger the hook
Tapable instance
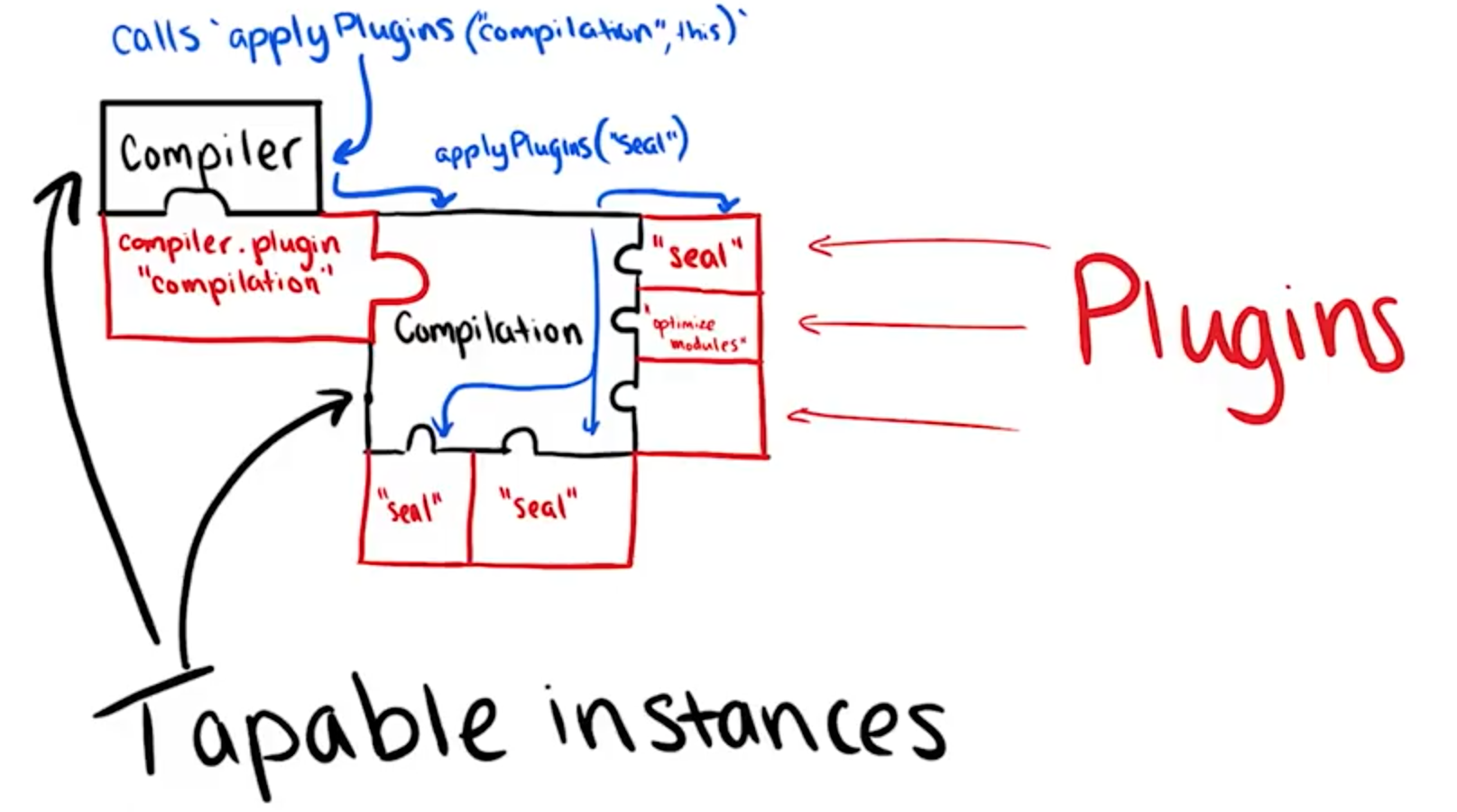
7 instances of Tapable
Compiler
exposed via node API
central dispatch
Start/stop
const webpack = require ('webpack'); const complier = webpack(<config>);
Compilation (dependency graph)
- created by the complier
- contains the dependency graph traversal algo
Resolver
- Finds the dependencies file
- Verifies the file exists
- turn partial path to abso path
- return the path, context, request
Module Factories
- Takes resolved request
- collect the source
- Returns Module object
Parser
- Convert a module object turns to AST tree
- finds all require/import and turn to dependency’s
{Template}
- Binding data with view/module
- config props
- Dep graph state
- template jsx
- Chunk ->module ->dependncies
- render() to bundle.js
How webpack builds the dependency graph
the complier reads the options and create compilations
after the resolver verfied these files, returns the normal modules
the parser, if non-js pass it to the loader, if it’s JS attach the dependencies to the module after resolved them
use template and module factore to remove/replace the require/import to run the app on browser
HOW A MODULE GETS TO THE BROWSER /w webpack
Cache-loader
Webpack-dev-middleware
Credits
all images in this post originats from the slides of this talk Sean Larkin’s talk Everything’s a Plugin: Understanding Webpack From the Inside Out at JS Kongress 2019