Disclaimer
This article is originally based on my understanding of this concept. No guarantee on the accuracy.π
Since the root and node share similar structure, itβs natural to use recursion to solve relative problem.
Also, iteration can also be applied. The key would be which kind of data structure to store the data of a tree. Normally, a queue(FIFO) or a stack(FILO) depeding on the traversal order.
Binary Tree in javascriptοΌ
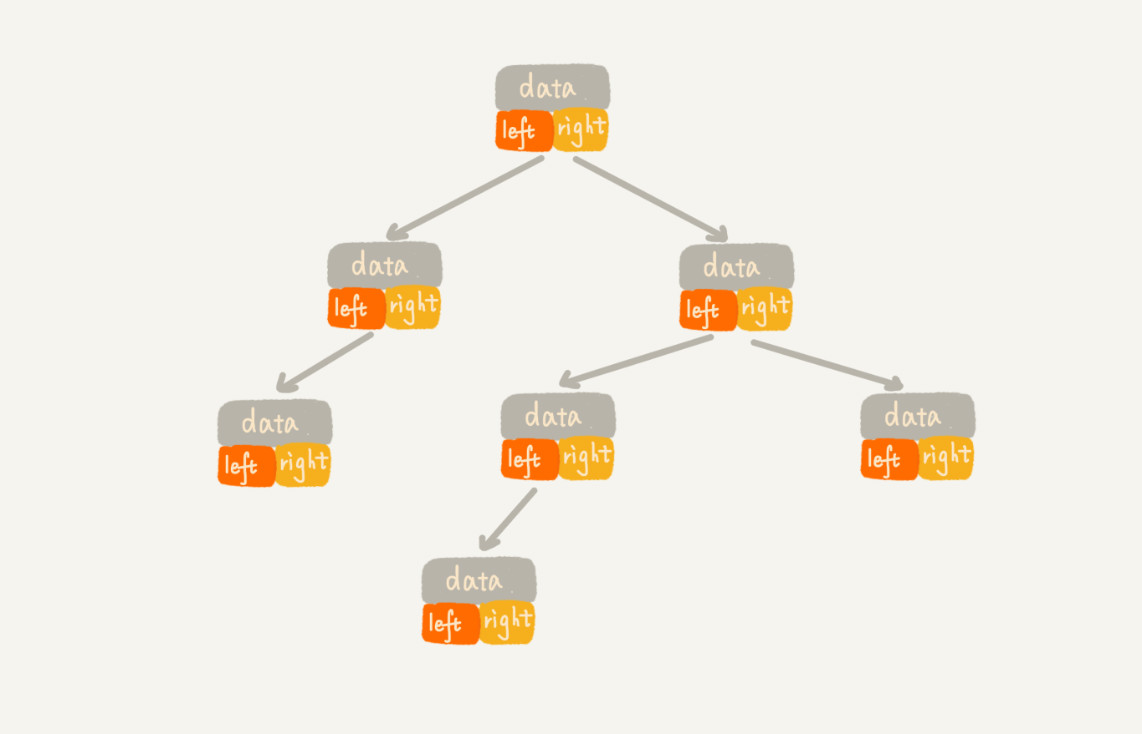
1 | function BinaryTree() { |
Or using an array to store the elements of the tree where the root node is stored at index 1 not 0.
For complete binary tree, if the index of an element (eg, D, i = 4). Its left child node will be stored in 2*i
(H,at 8), and its right childe node will be stored in2 * i-1
, its root node will be stored at Math.floor( i/2)
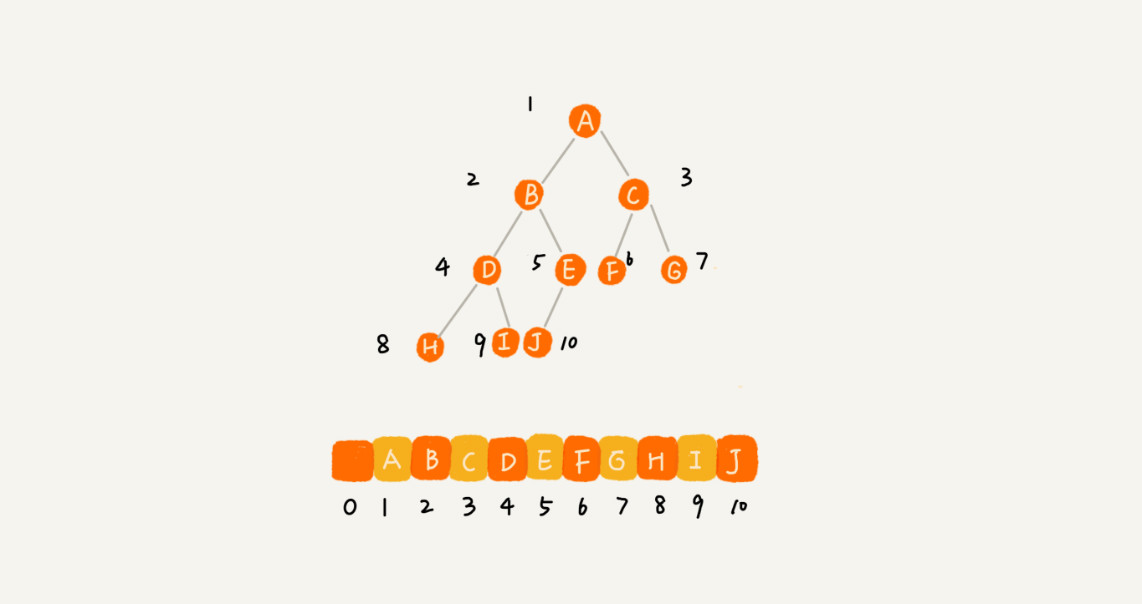
Traversal of tree
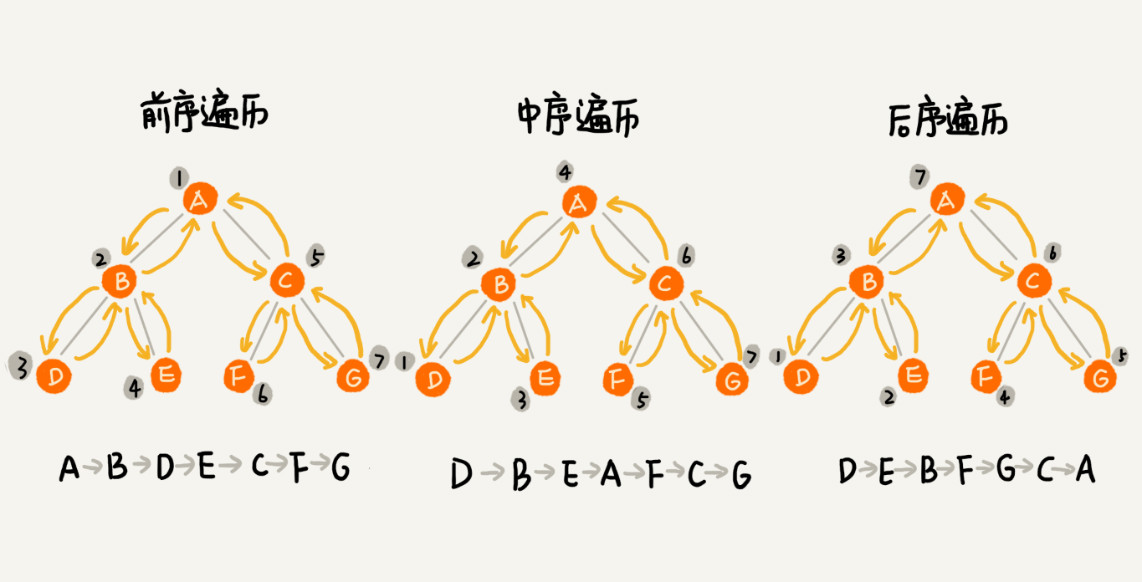
Depth-first | Breath-first |
---|---|
Preorder | Level-order |
Inorder | |
Postorder |
Preorder (root -> left node ->right node
Recursion:
1 | var preorderTraversal = function (root) { |
*Iteration: *
version 1:
1 | const preorderTraversal = (root) => { |
version 2:
1 | var preorderTraversal = function (root) { |
Inorder left node -> root -> right node
Recursion:
1 | var inorderTraversal = function (root) { |
Iteration:
General idea: Using a stack to record the iteration.
- If current node has left child node, push it to the stack, and move the current pointer to the left child node.
- If the current node dosenβt have left node (either only has right node or dosenβt have any child), obtain the previous node (the root of the current node) by poping the stack and push into the result. Move the current to the right of the previous node.
- The exit point is when the satck is empty and current node is pointing to null.
Version 1:
Left && push into the stack π <<ββββββββββββββββββββββββββββο½
!left && push into result and pop out from the stack mark the left child as visited(null) π ο½
right && push into the stack πββββββββββββββββββββββββββββββο½
1 | var inorderTraversal = function (root) { |
Version 2:
1 | var inorderTraversal = function(root) { |
Postorder left node -> right node->root
*Recursion: *
1 | var postOrder(root){ |
Iteration: (the reverse of preorder traversal)
Version1:
1 | const postorderTraversal = (root) => { |
Version 2:
var postorderTraversal = function (root) { var result = []; var stack = []; var p = root; while (stack.length != 0 || p != null) { if (p != null) { stack.push(p); result.unshift(p.val); // Reverse the process of preorder p = p.right; // Reverse the process of preorder } else { var node = stack.pop(); p = node.left; // Reverse the process of preorder } } return result; };
Summary:


