Type Conversion
Primitive
JavaScript is a loosely typed or a dynamic language. Variables in JavaScript are not directly associated with any particular value type, and any variable can be assigned (and re-assigned) values of all types:
There are 6 primitive data types: string, number, bigint, boolean, undefined, and symbol.
There also is null, which is seemingly primitive, but indeed is a special case for every Object: and any structured type is derived from null by the Prototype Chain.
Except for null and undefined, all primitive values have object equivalents that wrap around the primitive values:
String/ Number /BigInt /Boolean / Symbol
The wrapper’s valueOf() method returns the primitive value.
Explicit coercion
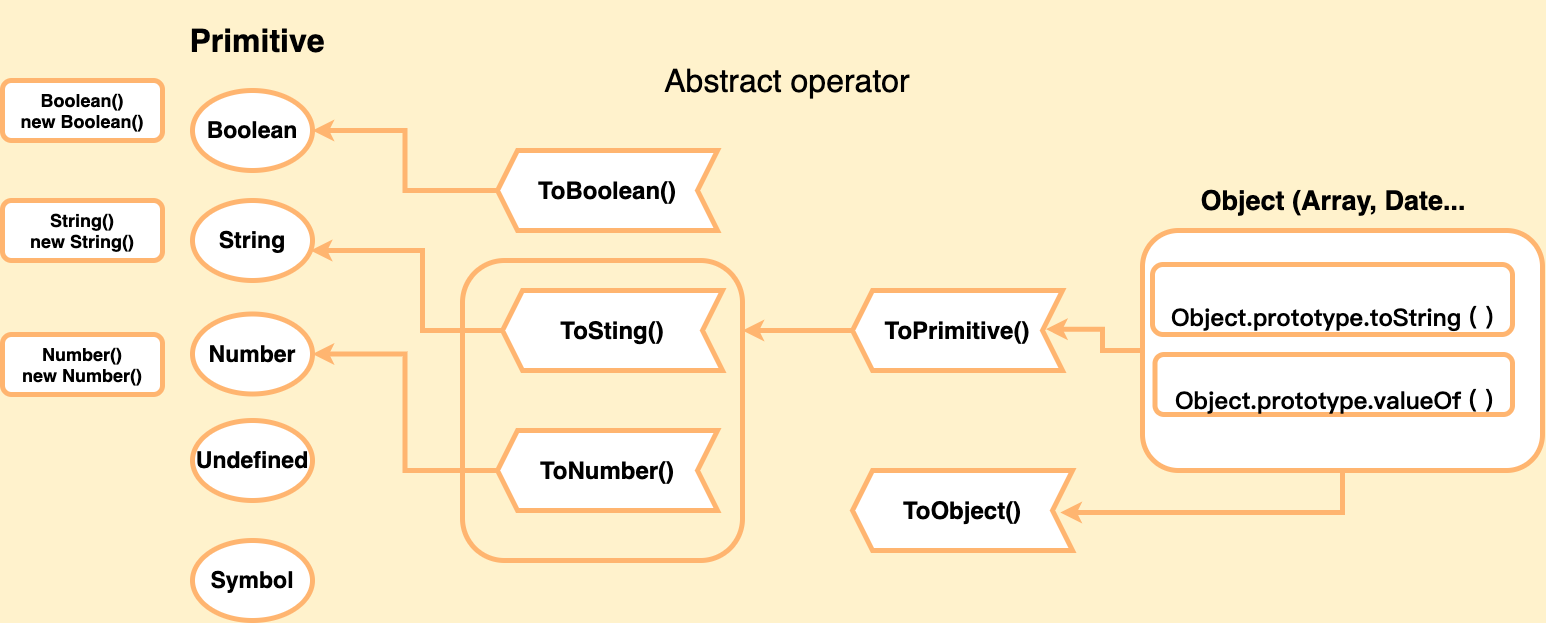
Boolean()
Primitive to Boolean
1 | console.log(Boolean()) // false |
Number()
When Number is called as a function rather than as a constructor, it performs a type conversion.
Returns a Number value (not a Number object) computed by ToNumber(value)
if value was supplied, else returns +0.
toNumber()
Type | Result |
---|---|
Undefined | NaN |
Null | +0 |
Boolean | true value-> 1 false value-> +0 |
Number | same number |
String |
1 | console.log(Number()) // +0 |
1 | console.log(parseInt("3 abc")) // 3 |
String()
When String
is called as a function rather than as a constructor, it performs a type conversion.
Returns a String value (not a String object) computed by ToString(value)
. If value is not supplied, the empty String “” is returned.
toString()
Type | Result |
---|---|
Undefined | “undefined” |
Null | “null” |
Boolean | true value-> “true” false value ->”false” |
Number | |
String | Same |
1 | console.log(String()) // 'empty string' |
Primitive type to reference type
String()、Number() and Boolean() constructor with new
keyword
1 | var a = 1; |
primitive to boolean
1 | console.log(Boolean(new Boolean(false))) // true |
Argument Type | Result |
---|---|
Undefined | Throw a TypeError exception. |
Null | Throw a TypeError exception. |
Object to string and number
first converts to primitive using toPrimitive, then convert to sting/number using ToString/ToNumber
Object.prototype
{
toString: ƒ toString()
valueOf: ƒ valueOf()
….
}let a ={}
a.valueOf= function(){ return ‘run this method’}
1 | let a ={a:'1'} |
1 | console.log(({}).toString()) // [object Object] |
Implicit coercion
(👆The tricky part)
Abstract Equality Comparison (==
)
Operand B | |||||||
---|---|---|---|---|---|---|---|
Undefined | Null | Number | String | Boolean | Object | ||
Operand A | Undefined | true |
true |
false |
false |
false |
false |
Null | true |
true |
false |
false |
false |
false |
|
Number | false |
false |
A === B |
A === ToNumber(B) |
A === ToNumber(B) |
A == ToPrimitive(B) |
|
String | false |
false |
ToNumber(A) === B |
A === B |
ToNumber(A) === ToNumber(B) |
A == ToPrimitive(B) |
|
Boolean | false |
false |
ToNumber(A) === B |
ToNumber(A) === ToNumber(B) |
A === B |
ToNumber(A) == ToPrimitive(B) |
|
Object | false |
false |
ToPrimitive(A) == B |
ToPrimitive(A) == B |
ToPrimitive(A) == ToNumber(B) |
A === B |
To primitive:
ToPrimitive(A)
attempts to convert its object argument to a primitive value, by attempting to invoke varying sequences of A.toString
and A.valueOf
methods on A
.
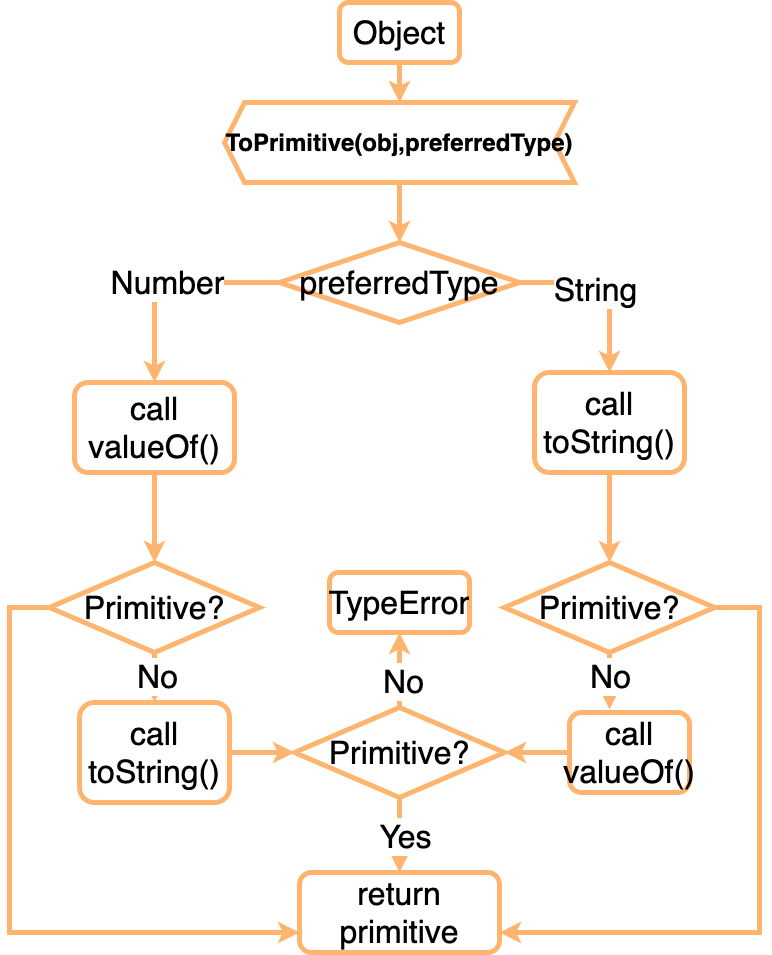
the piority of comparison type is
Number > String >Boolean>Obj
if one side is number, then convert to number; else if one side is boolean, convert to number
true=='2'//false true->Number ->1 '2'->Number ->2 false=='a'//false true='a'//false 'a'->Number->NaN <!--8-->
1 | [] == ![] //true |
‘+’ operartor
1 | var a = 21; |
|| && !
The value produced by a &&
or ||
operator is not necessarily of type Boolean. The value produced will always be the value of one of the two operand expressions.
1 | let A = ''; |
Ref: