Disclaimer
This article is originally based on my understanding of this concept. No guarantee on the accuracy.😅
Throttle
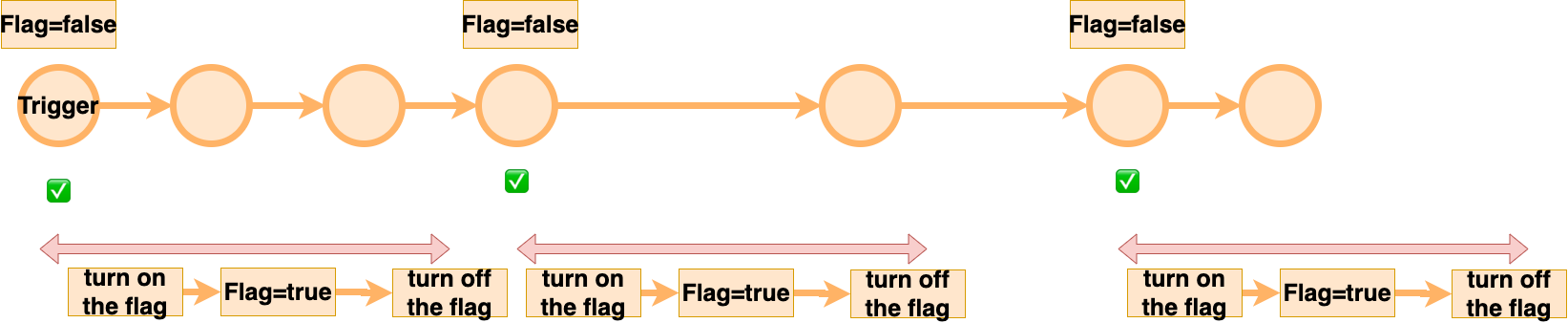
Throttle is used to limit how often a function can be called, though throttle is simpler to implement
Using a flag to record the state: within or exceed the time limit
Use case: during scrolling, resizing
1 | const throttle = (fn, limit) => { |
Debounce
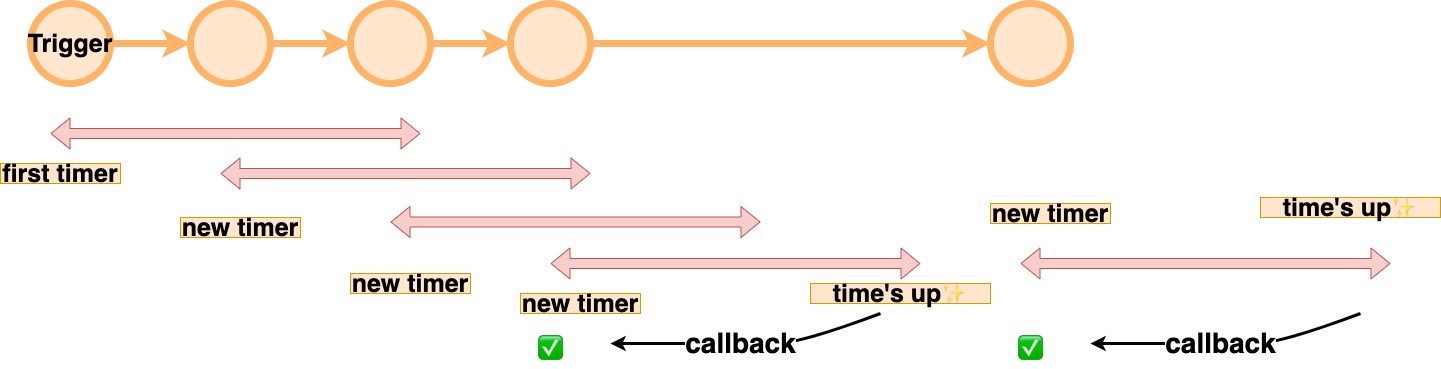
A debounced function is called only once in a given period, delay milliseconds after its last invocation (the timer is reset on every call).
use case: auto search
1 | const debounce = (fn, limit) => { |